Table of Contents
State management is an essential aspect of modern web development, especially when building dynamic and interactive user interfaces with React.js.
According to a survey by Stack Overflow in 2023, React.js remains one of the most popular frameworks among developers, with over 42% of respondents using it regularly.
This widespread adoption underscores the need for effective state management strategies to maintain performance and ensure a seamless user experience. As applications grow in complexity, managing state effectively becomes paramount to avoid common pitfalls such as unnecessary re-renders and tangled component hierarchies.
Advanced state management techniques are essential for developers aiming to build robust, scalable, and high-performance React applications. This guide dives deep into advanced state management methods, providing you with the knowledge and tools to handle state efficiently and effectively.
Whether you’re building a small project or a large-scale application, mastering these techniques will significantly enhance your development workflow.
Understanding State Management in React.js
State management refers to the handling of the application state. The data changes over time and needs to be tracked to provide an interactive experience. In React.js, the state is typically managed at the component level, but as applications grow, managing state across multiple components can become challenging.
# Why is State Management Important in React.js?
State management is crucial in React.js applications for several reasons
- Predictability: Proper state management ensures that your application behaves consistently.
- Debugging: With a well-defined state management strategy, tracking and fixing bugs becomes easier.
- Performance: Efficient state management can significantly improve application performance.
- Scalability: Managing state effectively is essential for scaling your application as it grows in complexity and size.
React.js State Management Techniques
# Local State Management
Local state management involves managing state within individual components using the useState hook. This is suitable for simple applications or components with limited state management needs.
jsx
import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); return ( <div> <wp-p>Count: {count}</wp-p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> ); }
# Lifting State Up
When multiple components need to share the same state, the state can be lifted up to the nearest common ancestor component. This approach ensures that all components have access to the same state.
jsx
import React, { useState } from 'react'; function ParentComponent() { const [sharedState, setSharedState] = useState('Hello World'); return ( <div> <ChildComponent1 sharedState={sharedState} /> <ChildComponent2 setSharedState={setSharedState} /> </div> ); } function ChildComponent1({ sharedState }) { return <wp-p>{sharedState}</wp-p>; } function ChildComponent2({ setSharedState }) { return <button onClick={() => setSharedState('Hello React')}>Change State</button>; }
Advanced State Management in React.js
# React Context API
The Context API is a powerful feature in React.js that allows you to share state across the entire application or specific parts of it without prop drilling. It’s particularly useful for global state management in React.js.
jsx
import React, { createContext, useContext, useState } from 'react'; const MyContext = createContext(); function App() { const [state, setState] = useState('Initial State'); return ( <MyContext.Provider value={{ state, setState }}> <ChildComponent /> </MyContext.Provider> ); } function ChildComponent() { const { state, setState } = useContext(MyContext); return ( <div> <wp-p>{state}</wp-p> <button onClick={() => setState('New State')}>Change State</button> </div> ); }
# Redux
Redux is a popular library for managing global state in React.js applications. It provides a centralized store for state, making state management predictable and easier to debug.
Key Concepts of Redux
- Store: The centralized state container.
- Actions: Plain objects representing events that change the state.
- Reducers: Pure functions that take the current state and an action, and return a new state.
- Dispatch: A function to send actions to the store.
Setting Up Redux in a React Application
jsx
// actions.js export const increment = () => ({ type: 'INCREMENT' }); // reducer.js const initialState = { count: 0 }; function counterReducer(state = initialState, action) { switch (action.type) { case 'INCREMENT': return { count: state.count + 1 }; default: return state; } } export default counterReducer; // store.js import { createStore } from 'redux'; import counterReducer from './reducer'; const store = createStore(counterReducer); export default store; // App.js import React from 'react'; import { Provider, useDispatch, useSelector } from 'react-redux'; import store, { increment } from './store'; function Counter() { const count = useSelector(state => state.count); const dispatch = useDispatch(); return ( <div> <wp-p>Count: {count}</wp-p> <button onClick={() => dispatch(increment())}>Increment</button> </div> ); } function App() { return ( <Provider store={store}> <Counter /> </Provider> ); } export default App;
# React Query
React Query is one of the powerful tools for managing server-side state in React.js applications. It simplifies fetching, caching, and synchronizing server data with your UI.
jsx
import React from 'react'; import { useQuery } from 'react-query'; function fetchPosts() { return fetch('https://jsonplaceholder.typicode.com/posts').then(res => res.json()); } function Posts() { const { data, error, isLoading } = useQuery('posts', fetchPosts); if (isLoading) return <wp-p>Loading...</wp-p>; if (error) return <wp-p>Error loading posts</wp-p>; return ( <ul> {data.map(post => ( <li key={post.id}>{post.title}</li> ))} </ul> ); } export default Posts;
Also Read:- How Much Does It Cost To Hire A React JS Developer?
# MobX
MobX is another popular state management library that emphasizes simplicity and reactivity. It allows you to manage state in an intuitive way, making it easy to track and react to changes.
Key Concepts of Redux
- Observable State: State that MobX tracks for changes.
- Actions: Functions that modify the state.
- Computed Values: Values derived from the state.
- Reactions: Functions that react to state changes.
Setting Up MobX in a React Application
jsx
import React from 'react'; import { observer } from 'mobx-react'; import { makeAutoObservable } from 'mobx'; class CounterStore { count = 0; constructor() { makeAutoObservable(this); } increment() { this.count++; } } const counterStore = new CounterStore(); const Counter = observer(() => { return ( <div> <wp-p>Count: {counterStore.count}</wp-p> <button onClick={() => counterStore.increment()}>Increment</button> </div> ); }); function App() { return ( <div> <Counter /> </div> ); } export default App;
React State Management Best Practices
- Keep State Local Where Possible: Whenever possible, keep state local to the component that needs it. This minimizes the complexity of your application and makes it easier to manage.
- Use the Context API for Global State: For global state, the Context API is a lightweight solution that integrates seamlessly with React. It avoids the overhead of external libraries like Redux when the state management requirements are simple.
- Leverage Redux for Complex State: When your application grows in complexity, consider using Redux for state management. Its robust ecosystem and middleware support make it suitable for large-scale applications.
- Optimize Performance with React Query: For server-side state management, React Query is a great choice. It handles caching, synchronization, and background updates efficiently, improving the performance and user experience of your application.
- Separate UI and State Logic: Keep your state management logic separate from your UI components. This makes your components more reusable and your state management more maintainable.
- Use Middleware for Side Effects: When using Redux, leverage middleware like redux-thunk or redux-saga to handle side effects such as asynchronous actions. This keeps your action creators pure and makes your codebase cleaner.
- Implement Error Handling: Robust error handling is essential for a smooth user experience. Ensure that your state management strategy includes mechanisms for catching and handling errors gracefully.
- Write Unit Tests: Writing unit tests for your state management logic helps catch bugs early and ensures that your state behaves as expected. Tools like Jest and React Testing Library are great for this purpose.
Summing Up
Effective state management is essential for building scalable React.js applications. By mastering both basic and advanced state management techniques, you can tackle the challenges of managing the state in complex applications. Whether you’re using the Context API for the global state, Redux for the complex state, MobX for simplicity, or React Query for the server-side state, understanding and applying these techniques will significantly improve your development process.
If you need professional help with your React.js projects, get assistance from professional React.js development services providers. Expert developers can ensure your application is built with best practices, delivering a high-quality user experience. Remember, good state management is key to the success of your React.js applications. With the right approach, you can maintain performance, scalability, and ease of maintenance, ensuring a positive experience for your users.
State management in React.js is a multi-faceted aspect of development. By exploring various state management strategies and choosing the right tools for your specific needs, you can create applications that are not only functional but also efficient and maintainable. Keep learning and experimenting with different approaches to find the best fit for your projects. And remember, whether it’s local state, global state, or server-side state, each has its place and purpose in the React ecosystem.
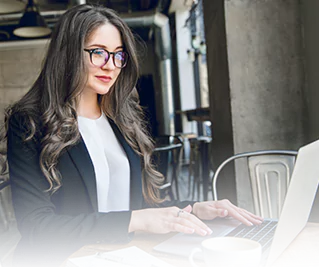