Table of Contents
Flash messages are an essential part of web applications as they provide a way to give feedback to users. They are short, informative messages that inform users about the outcome of their actions, such as successful form submissions or errors. In Node.js, you can easily implement flash messages using the connect-flash module. This guide will walk you through the steps required to set up and display flash messages in a Node.js application using connect-flash.
What is connect-flash?
connect-flash is a middleware for Node.js that allows you to store messages in the session to be displayed after a redirect. It is often used with Express.js, a popular web framework for Node.js. This module is especially useful for displaying success or error messages after form submissions or other user actions, making it a valuable tool for businesses offering Node.js development services.
Prerequisites
Before we begin, make sure you have the following installed:
- Node.js
- npm (Node Package Manager)
- Express.js (if not, you can install it using npm)
Step 1: Set Up Your Node.js Application
First, create a new directory for your project and navigate into it:
mkdir flash-messages-demo cd flash-messages-demo
Initialize a new Node.js project:
npm init -y
Install the necessary packages:
npm install express express-session connect-flash ejs
Step 2: Create the Basic Express Application
Create a file named “app.js” and set up a basic Express server:
const express = require('express'); const session = require('express-session'); const flash = require('connect-flash'); const app = express(); app.set('view engine', 'ejs'); app.use(express.urlencoded({ extended: true })); app.use(session({ secret: 'secret', resave: false, saveUninitialized: true, })); app.use(flash()); app.get('/', (req, res) => { res.render('index', { message: req.flash('message') }); }); app.post('/submit', (req, res) => { const { name } = req.body; if (name) { req.flash('message', 'Form submitted successfully!'); } else { req.flash('message', 'Form submission failed. Please try again.'); } res.redirect('/'); }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
In this setup:
- We require the necessary modules (“express”, “express-session”, and “connect-flash”).
- We set the view engine to EJS for rendering templates.
- We set up the session middleware to handle session data.
- We use the “connect-flash” middleware to store flash messages.
- We create two routes: a GET route for the home page and a POST route for form submission.
Step 3: Create the Views
Create a folder named “views” and inside it, create a file named “index.ejs”:
<!DOCTYPE html> <html> <head> <title>Flash Messages Demo</title> </head> <body> <h1>Flash Messages Demo</h1> <% if (message.length > 0) { %> <div><%= message %></div> <% } %> <form action="/submit" method="POST"> <input type="text" name="name" placeholder="Enter your name"> <button type="submit">Submit</button> </form> </body> </html>
This simple HTML form allows users to submit their name. If a flash message exists, it will be displayed above the form.
Step 4: Handle Flash Messages
The flash messages are stored in the session and will be removed after being displayed. This behavior makes flash messages perfect for showing temporary notifications.
Step 5: Test Your Application
Start your application:
node app.js
Open your browser and navigate to “http://localhost:3000”. You should see a form where you can enter your name and submit it. Depending on whether you enter a name or not, you will see a success or error message displayed above the form.
# Advanced Usage of Flash Messages
Display Multiple Types of Messages
In many applications, you may need to display different types of messages, such as success, error, or informational messages. You can achieve this by using different keys for each type of message.
Modify your “app.js” file as follows:
app.post('/submit', (req, res) => { const { name } = req.body; if (name) { req.flash('success', 'Form submitted successfully!'); } else { req.flash('error', 'Form submission failed. Please try again.'); } res.redirect('/'); }); app.get('/', (req, res) => { res.render('index', { success: req.flash('success'), error: req.flash('error'), }); });
Update your “index.ejs” file to display different types of messages:
<!DOCTYPE html> <html> <head> <title>Flash Messages Demo</title> </head> <body> <h1>Flash Messages Demo</h1> <% if (success.length > 0) { %> <div style="color: green;"><%= success %></div> <% } %> <% if (error.length > 0) { %> <div style="color: red;"><%= error %></div> <% } %> <form action="/submit" method="POST"> <input type="text" name="name" placeholder="Enter your name"> <button type="submit">Submit</button> </form> </body> </html>
Persisting Flash Messages Across Redirects
By default, flash messages are removed from the session after they are displayed. If you need to persist them across multiple redirects, you can manually reassign them to the session.
Modify your “app.js” file as follows:
app.use((req, res, next) => { res.locals.success = req.flash('success'); res.locals.error = req.flash('error'); next(); }); app.get('/', (req, res) => { res.render('index'); });
Now, the flash messages will persist in res.locals and can be accessed in your views without needing to pass them explicitly.
Conclusion
Implementing flash messages in your Node.js applications can significantly improve the user experience by providing clear and immediate feedback on their actions. Whether you are displaying success messages after form submissions or error notifications when something goes wrong, the “connect-flash” module offers a straightforward and effective solution.
For businesses looking to build robust and user-friendly Node.js applications, Shiv Technolabs offers top-notch Node.js development services in Turkey. Our expert team delivers applications developed to the highest standards, incorporating best practices for features like flash messaging and more. Partner with Shiv Technolabs to bring your project to life with exceptional Node.js development services in Turkey.
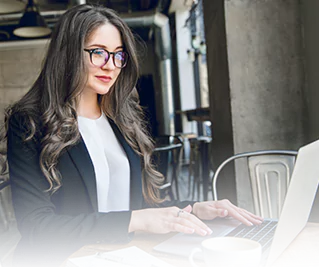